scene sceneobjects images/textures/animated sprites sounds camera lights bounding box direction/angle animation & easing functions sphere collision bounds collision function abs(a) { return Math.abs(a); } function length(a) { return Math.sqrt(a.x*a.x + a.y*a.y + a.z*a.z); } function distance(a, b) { return Math.sqrt((a.x - b.x) * (a.x - b.x) + (a.y - b.y) * (a.y - b.y) + (a.z - b.z) * (a.z - b.z)); } var p1 = { x: 20, y: 20 }; var p2 = { x: 40, y: 40 }; // angle in radians var angleRadians = Math.atan2(p2.y - p1.y, p2.x - p1.x); // angle in degrees var angleDeg = Math.atan2(p2.y - p1.y, p2.x - p1.x) * 180 / Math.PI; // http://onedayitwillmake.com/blog/2011/09/getting-the-angle-between-two-3d-points/ function() { // Make up two vectors var vectorA = new Vector3(5, -20, -14); var vectorB = new Vector3(-1, 3, 2); // Store some information about them for below var dot = vectorA.dot(vectorB); var lengthA = vectorA.length(); var lengthB = vectorB.length(); // Now to find the angle var theta = Math.acos( dot / (lengthA * lengthB) ); // Theta = 3.06 radians or 175.87 degrees // But what can you do with it? } function now() { return (new Date()).getTime() / 1000.0; } /// Check intersections / collisions /// https://developer.mozilla.org/en-US/docs/Games/Techniques/3D_collision_detection function isPointInsideAABB(point, box) { return (point.x >= box.minX && point.x <= box.maxX) && (point.y >= box.minY && point.y <= box.maxY) && (point.z >= box.minY && point.z <= box.maxZ); } function intersectCubes(a, b) { return (a.minX <= b.maxX && a.maxX >= b.minX) && (a.minY <= b.maxY && a.maxY >= b.minY) && (a.minZ <= b.maxZ && a.maxZ >= b.minZ); } function intersectSpheres(a, b) { // we are using multiplications because it's faster than calling Math.pow var distance = Math.sqrt((a.x - b.x) * (a.x - b.x) + (a.y - b.y) * (a.y - b.y) + (a.z - b.z) * (a.z - b.z)); return distance < (a.radius + b.radius); } function intersectSphereBox(sphere, box) { // get box closest point to sphere center by clamping var x = Math.max(box.minX, Math.min(sphere.x, box.maxX); var y = Math.max(box.minY, Math.min(sphere.y, box.maxY); var z = Math.max(box.minZ, Math.min(sphere.z, box.maxZ); // this is the same as isPointInsideSphere var distance = Math.sqrt((x - sphere.x) * (x - sphere.x) + (y - sphere.y) * (y - sphere.y) + (z - sphere.z) * (z - sphere.z)); return distance < sphere.radius; } function doStep(factor) { objects.forEach(function(object) { object.prevPosition = object.position; if (object.velocity) object.position += (factor * object.velocity); if (object.acceleration) object.velocity += (factor * object.acceleration); if (object.friction) object.velocity -= (factor * object.friction); object.collisionCheck(); }; } var speed = 1; var prevTime = null; function step(timestamp) { if (!prevTime) { prevTime = now(); } var elapsed = now() - prevTime; factor = elapsed * speed; if (factor > 0) { doStep(factor); } window.requestAnimationFrame(step); } window.requestAnimationFrame(step);
https://www.codeproject.com/Articles/43751/Battlefield-Simulator
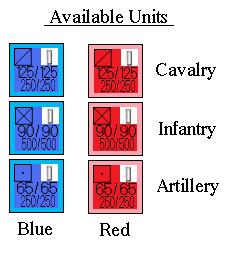
61700cookie-checkJavascript Game functions