// ISO 6346 // does NOT validate the "Check Digit" private static Regex containerNumberRegex = new Regex("^[a-zA-Z]{3}[uUjJzZ]{1}[0-9]{7}$"); public static bool IsValidContainerNumber(string text) => containerNumberRegex.IsMatch(text); IsValidContainerNumber(" MEDU8707688 ".Trim()) ////////////////////////////// // Code to get Check digit ////////////////////////////// private static string CharacterValues = "0123456789A_BCDEFGHIJK_LMNOPQRSTU_VWXYZ"; // skip 11th values // short Linq version private static int GetCheckDigit(string containerNumber) { var sum = containerNumber.Select((c, i) => CharacterValues.IndexOf(c) * Math.Pow(2, i)).Sum(); return (int)(sum - (Math.Floor(sum / 11.0) * 11.0)); } // comprehensive version private static int GetCheckDigit(string containerNumber) { var sum = 0.0; for (int i = 0; i < containerNumber.Count(); i++) { char c = containerNumber[i]; var power = Math.Pow(2, i); var charValue = CharacterValues.IndexOf(c); Console.WriteLine($"{i}: [{c}] {power,4} * {charValue,4} = {power*charValue,4}"); sum += CharacterValues.IndexOf(c) * power; } var rest = Math.Floor(sum / 11.0) * 11.0; Console.WriteLine($"sum-rest: {sum} - {rest} = {sum - rest}"); return (int)Math.Floor(sum - rest); } void Main() { Console.WriteLine(GetCheckDigit("CSQU305438")); } /* OUTPUT: 0: [C] 1 * 13 = 13 1: [S] 2 * 30 = 60 2: [Q] 4 * 28 = 112 3: [U] 8 * 32 = 256 4: [3] 16 * 3 = 48 5: [0] 32 * 0 = 0 6: [5] 64 * 5 = 320 7: [4] 128 * 4 = 512 8: [3] 256 * 3 = 768 9: [8] 512 * 8 = 4096 sum-rest: 6185 - 6182 = 3 3 */
What is ISO 6346 (wikipedia definition)
ISO 6346 is an international standard covering the coding, identification and marking of intermodal (shipping) containers used within containerized intermodal freight transport.The standard establishes a visual identification system for every container that includes a unique serial number (with check digit), the owner, a country code, a size, type and equipment category as well as any operational marks. The standard is managed by the International Container Bureau (BIC).
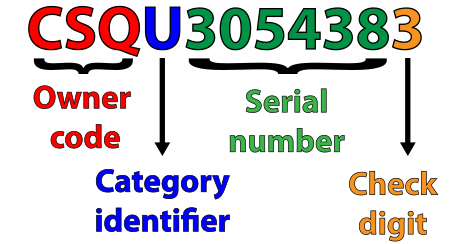
Step | Container number | Total |
---|---|---|
C S Q U 3 0 5 4 3 8 | ||
1 | 13 30 28 32 3 0 5 4 3 8 | |
x x x x x x x x x x | ||
2 | 1 2 4 8 16 32 64 128 256 512 | |
= = = = = = = = = = | ||
13 60 112 256 48 0 320 512 768 4096 | ||
3 | Sum all results from (2) | 6185 |
4 | Divide (3) by 11 (remainder discarded) | 562 |
5 | Multiply (4) by 11 | 6182 |
6 | (3) minus (5) = Check Digit: | 3 |
Step 1
An equivalent numerical value is assigned to each letter of the alphabet, beginning with 10 for the letter A (11 and multiples thereof are omitted):
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
---|---|---|---|---|---|---|---|---|---|
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
A | B | C | D | E | F | G | H | I | J | K | L | M |
---|---|---|---|---|---|---|---|---|---|---|---|---|
10 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 23 | 24 |
N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
---|---|---|---|---|---|---|---|---|---|---|---|---|
25 | 26 | 27 | 28 | 29 | 30 | 31 | 32 | 34 | 35 | 36 | 37 | 38 |
Step 2
Each of the numbers calculated in step 1 is multiplied by 2position, where position is the exponent to base 2. Position starts at 0, from left to right.
Step 3
Sum up all results of Step above
Step 4
Round the result down towards zero i.e. make the result a whole number (integer)
Step 5
Divide them by 11
Step 6
Multiply the integer value by 11
Step 7
Subtract result of Step 3 from result of (6): This is the check digit.