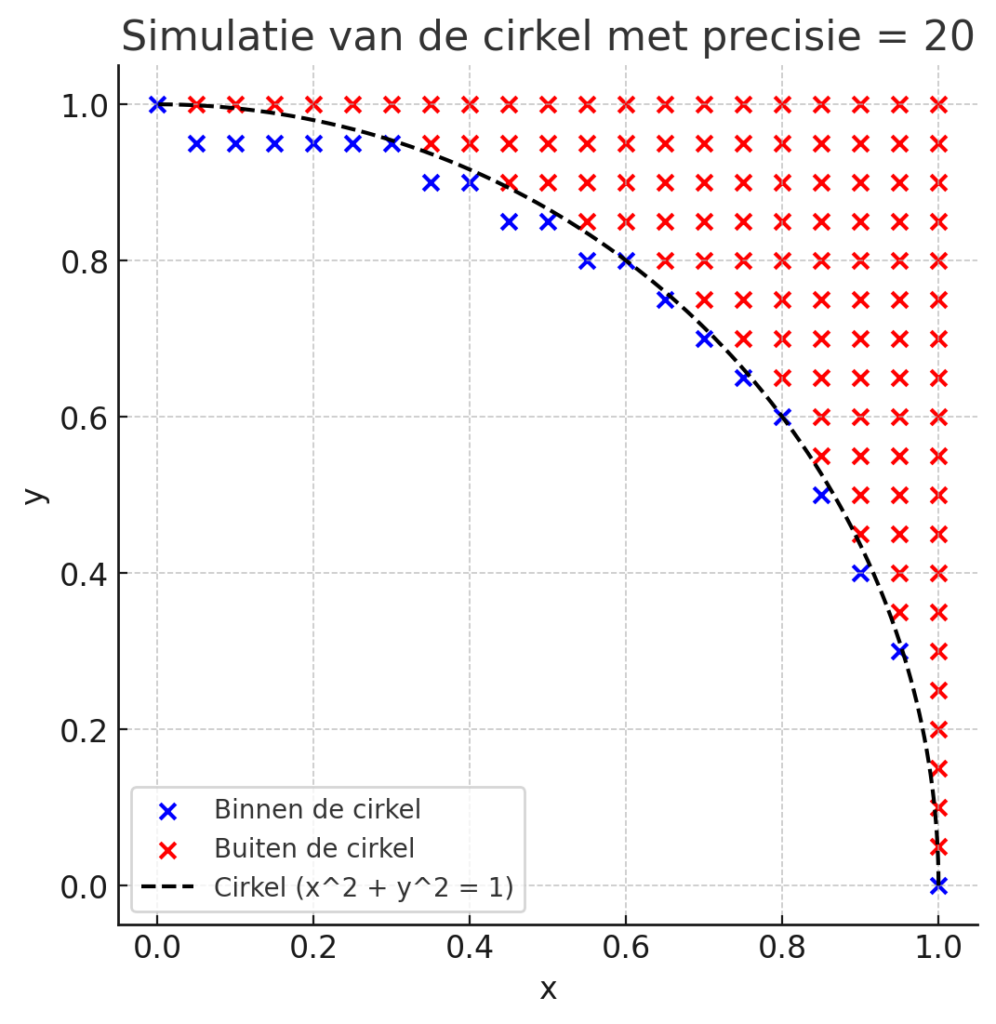
function calculatePi(precision) { let total = (precision + 1) * (precision + 1); // Inclusief alle punten let outside = 0; let xP, yP; // Loop alleen over de helft van de waarden van x (symmetrie gebruiken) for (let x = precision; x >= 0; x--) { xP = x / precision; for (let y = precision; y >= 0; y--) { if (x >= y) break; // Itereer alleen over de onderste helft van x yP = y / precision; if (xP * xP + yP * yP > 1.0) { outside++; } else { break; // Zodra een punt binnen de cirkel is gevonden, kunnen we stoppen } } } // Correcte telling van het aantal binnenliggende punten let inside = total - (outside * 2); // Bereken Pi return (inside / total) * 4; // De ratio van binnen tot totale punten, vermenigvuldigd met 4 } console.log('calcated = ', calculatePi(300000)); console.log('PI = ', Math.PI); console.log('355 / 113 = ', 355 / 113); console.log('1-9 = ', 3 + 4 / 28 - 1 / (790 + 5 / 6))
Gauss-Legendre algoritm
(() => { // Gauss-Legendre-algoritm function calculatePi(iterations) { let a = 1.0; let b = 1.0 / Math.sqrt(2); let t = 1.0 / 4.0; let p = 1.0; for (let i = 0; i < iterations; i++) { const a_next = (a + b) / 2.0; const b_next = Math.sqrt(a * b); t -= p * Math.pow(a - a_next, 2); p *= 2.0; a = a_next; b = b_next; } const pi = Math.pow(a + b, 2) / (4 * t); return pi; } const iterations = 5; // Slechts een paar iteraties nodig const piEstimate = calculatePi(iterations); console.log(`Geschatte waarde van Pi met ${iterations}`); console.log(`Waarde: ${piEstimate}`); console.log(`PI: ${Math.PI}`); })();
-- Gauss-Legendre-algoritme function calculatePi(iterations) local a = 1.0 local b = 1.0 / math.sqrt(2) local t = 1.0 / 4.0 local p = 1.0 for i = 1, iterations do local a_next = (a + b) / 2.0 local b_next = math.sqrt(a * b) t = t - p * math.pow(a - a_next, 2) p = p * 2.0 a = a_next b = b_next end local pi = math.pow(a + b, 2) / (4.0 * t) return pi end local iterations = 5 -- Slechts een paar iteraties nodig local piEstimate = calculatePi(iterations) print(string.format("Geschatte waarde van Pi met %d iteraties: %.15f", iterations, piEstimate)) print(string.format("Werkelijke waarde van Pi: %.15f", math.pi))
#r "nuget: NBitcoin, 7.0.37" using System; using NBitcoin; class Program { static void Main() { int precision = 100; // Aantal decimalen van π int iterations = 10; // Aantal iteraties van het Gauss-Legendre algoritme BigDecimal a = new BigDecimal(1, precision); BigDecimal b = BigDecimal.One / BigDecimal.Sqrt(new BigDecimal(2, precision), precision); BigDecimal t = new BigDecimal(0.25m, precision); BigDecimal p = BigDecimal.One; for (int i = 0; i < iterations; i++) { BigDecimal aNext = (a + b) / 2; b = BigDecimal.Sqrt(a * b, precision); t -= p * BigDecimal.Pow(a - aNext, 2); a = aNext; p *= 2; } BigDecimal pi = (a + b) * (a + b) / (t * 4); Console.WriteLine($"π ≈ {pi.ToString().Substring(0, precision + 2)}"); // Precision + 2 to include "3." } }
887100cookie-checkCalculate PI